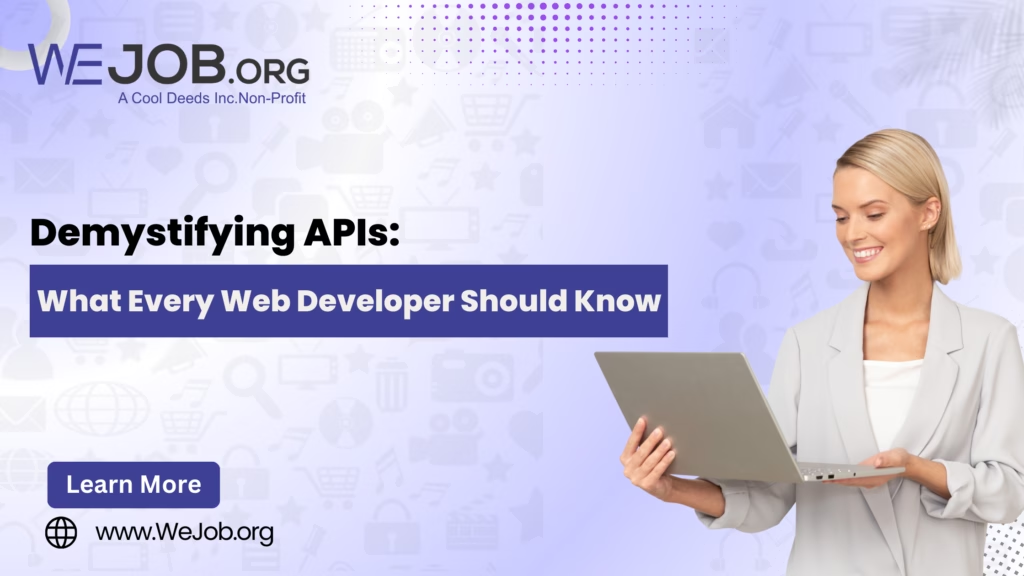
APIs (Application Programming Interfaces) are at the heart of modern web development, enabling seamless communication between different software systems. From online payments to fetching live weather updates, APIs simplify complex tasks and power today’s web applications.
This guide will break down the fundamentals of APIs, their types, how they work, and why they’re essential for web developers. By the end, you’ll understand how to integrate APIs into your projects effectively and overcome common challenges.
What Are APIs?
An API is like a digital intermediary that allows one application to communicate with another. Think of it as a waiter in a restaurant who takes your order (request), delivers it to the kitchen (server), and brings back your food (response).
Real-World Examples of APIs:
- A weather API fetches live weather data.
- A payment gateway API processes transactions on e-commerce platforms.
How Do APIs Work?
APIs operate through a request-and-response cycle:
Request: A client (e.g., browser or app) sends a request to the API, typically including:
- An endpoint (URL)
- Parameters (optional)
- Authentication credentials (e.g., API keys)
Processing: The API processes the request by accessing its server or database.
Response: The API returns the requested data or confirms the requested action, often in JSON or XML format.
Example:
Request:
GET https://api.weather.com/v1/current?city=NewYork
Response:
{
“city”: “New York”,
“temperature”: “12°C”,
“condition”: “Cloudy”
}
Types of APIs
APIs come in various flavors, each suited for specific purposes:
1. REST APIs (Representational State Transfer):
- Most widely used API type.
- Uses HTTP methods like GET, POST, PUT, DELETE.
- Returns lightweight JSON data.
2. SOAP APIs (Simple Object Access Protocol):
- More rigid and structured.
- Uses XML for data exchange.
- Often employed in enterprise-level systems requiring high security.
3. GraphQL APIs:
- Developed by Facebook.
- Allows clients to request exactly the data they need.
- Avoids over-fetching or under-fetching of data.
4. Webhooks:
- Sends data automatically when a specific event occurs.
- Used for real-time applications like payment notifications.
5. Open APIs:
- Publicly available, such as Google Maps API or Twitter API.
Why Are APIs Crucial for Web Developers?
APIs streamline development by enabling powerful integrations and providing pre-built functionalities. Here’s why they’re indispensable:
- Third-Party Integration: Simplifies adding features like payment gateways or social media logins.
- Reusability: Saves time by leveraging existing services.
- Scalability: Allows feature expansion without overloading codebases.
- Consistency: Standardizes interactions with systems.
- Access to Real-Time Data: Fetches live updates like stock prices or news feeds.
How to Work with APIs
1. Understand API Documentation
- Learn about endpoints, request methods, parameters, and response formats.
2. Manage Authentication
- Many APIs require API keys, OAuth tokens, or other authentication methods. Always keep these secure.
3. Test APIs
- Use tools like Postman or cURL to test endpoints and ensure they work as expected.
4. Integration in Code
- Use languages like JavaScript, Python, or PHP for integration.
Example (JavaScript):
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(‘Error:’, error));
5. Handle Errors Gracefully
- Always include error handling to manage issues like timeouts or incorrect parameters.
Example:
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
Overcoming Common Challenges
Rate Limits:
APIs often limit the number of requests per timeframe. Use caching to optimize usage.Data Format Issues:
Ensure proper handling of JSON or XML data.Authentication Errors:
Double-check API keys or tokens to avoid access issues.Versioning:
Stay updated with API changes to avoid breaking integrations.